24 code ES6 bạn nên biết để khắc phục các lỗi thực hành JavaScript
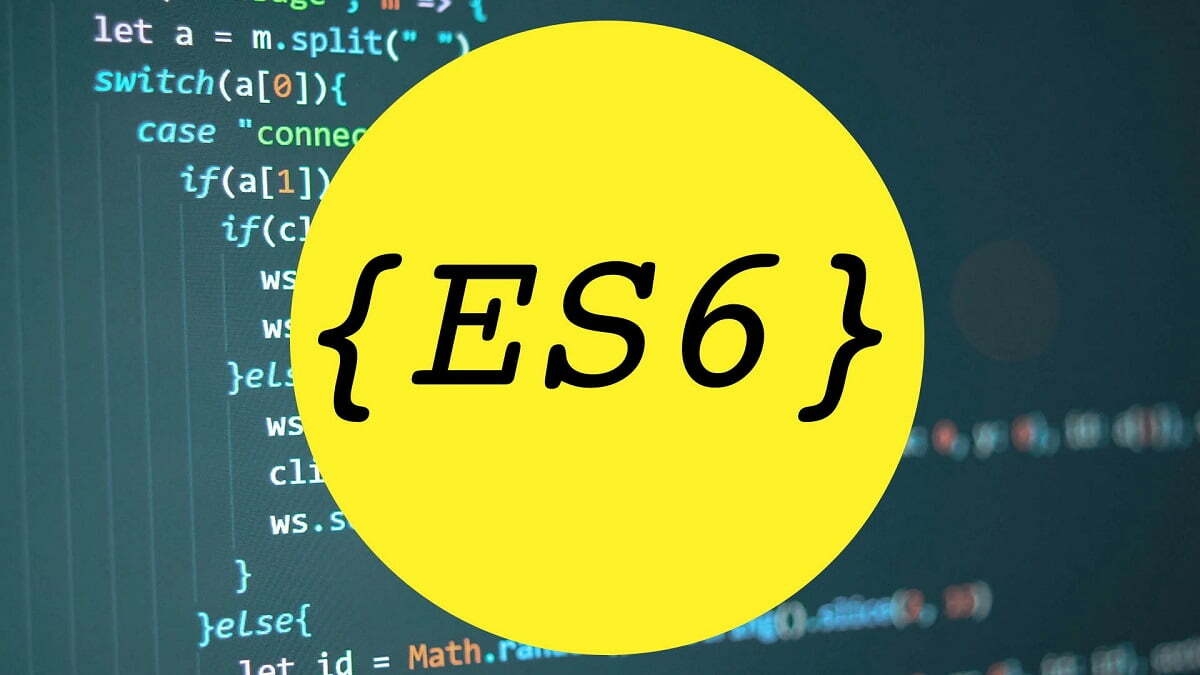
1. Cách để ẩn các phần tử bất kỳ trong JavaScript
const hide = (...el) => [...el].forEach(e => (e.style.display = 'none'));
// Example
hide(document.querySelectorAll('img')); // Hides all
elements on the page
2. Cách kiểm tra xem phần tử có chứa class nào đó hay không
const hasClass = (el, className) => el.classList.contains(className);
// Example
hasClass(document.querySelector('p.special'), 'special'); // true
3. Toggle Class
const toggleClass = (el, className) => el.classList.toggle(className);
// Example
toggleClass(document.querySelector('p.special'), 'special');
// The paragraph will not have the 'special' class anymore
4. Cách lấy vị trí cuộn của trang hiện tại
const getScrollPosition = (el = window) => ({
x: el.pageXOffset !== undefined ? el.pageXOffset : el.scrollLeft,
y: el.pageYOffset !== undefined ? el.pageYOffset : el.scrollTop
});
// Ví dụ
getScrollPosition(); // {x: 0, y: 200}
5. Cách cuộn mượt mà lên đầu trang
const scrollToTop = () => {
const c = document.documentElement.scrollTop || document.body.scrollTop;
if (c > 0) {
window.requestAnimationFrame(scrollToTop);
window.scrollTo(0, c - c / 8);
}
};
// Example
scrollToTop();
6. Cách để kiểm tra xem phần tử cha có chứa phần tử con không
const elementContains = (parent, child) => parent !== child && parent.contains(child); // Ví dụ elementContains(document.querySelector('head'), document.querySelector('title')); // true elementContains(document.querySelector('body'), document.querySelector('body')); // false
7. Cách để kiểm tra xem phần tử được chỉ định có hiển thị trong chế độ xem không
const elementIsVisibleInViewport = (el, partiallyVisible = false) => {
const { top, left, bottom, right } = el.getBoundingClientRect();
const { innerHeight, innerWidth } = window;
return partiallyVisible
? ((top > 0 && top < innerHeight) || (bottom > 0 && bottom < innerHeight)) &&
((left > 0 && left < innerWidth) || (right > 0 && right < innerWidth))
: top >= 0 && left >= 0 && bottom <= innerHeight && right <= innerWidth;
};
// Examples
elementIsVisibleInViewport(el); // (Không hiển thị đầy đủ)
elementIsVisibleInViewport(el, true); // (Hiển thị một phần)
8. Cách để tìm nạp tất cả các hình ảnh trong một phần tử
const getImages = (el, includeDuplicates = false) => {
const images = [...el.getElementsByTagName('img')].map(img => img.getAttribute('src'));
return includeDuplicates ? images : [...new Set(images)];
};
// Ví dụ
getImages(document, true); // ['image1.jpg', 'image2.png', 'image1.png', '...']
getImages(document, false); // ['image1.jpg', 'image2.png', '...']
9. Cách để biết được thiết bị là thiết bị di động hay máy tính để bàn / máy tính xách tay
const detectDeviceType = () =>
/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)
? 'Mobile'
: 'Desktop';
// Ví dụ
detectDeviceType(); // "Mobile" hoặc "Desktop"
10. Cách để có được URL hiện tại
const currentURL = () => window.location.href;
// Example
currentURL(); // 'https://google.com'
11. Cách để tạo một đối tượng chứa các tham số của URL hiện tại
const getURLParameters = url =>
(url.match(/([^?=&]+)(=([^&]*))/g) || []).reduce(
(a, v) => ((a[v.slice(0, v.indexOf('='))] = v.slice(v.indexOf('=') + 1)), a),
{}
);
// Examples
getURLParameters('http://url.com/page?n=Adam&s=Smith'); // {n: 'Adam', s: 'Smith'}
getURLParameters('google.com'); // {}
12. Cách để mã hóa một tập hợp các phần tử form dưới dạng một đối tượng
const formToObject = form =>
Array.from(new FormData(form)).reduce(
(acc, [key, value]) => ({
...acc,
[key]: value
}),
{}
);
// Example
formToObject(document.querySelector('#form')); // { email: 'test@email.com', name: 'Test Name' }
13. Cách để lấy một tập các thuộc tính được chỉ định bởi các bộ chọn đã cho từ một đối tượng
const get = (from, ...selectors) =>
[...selectors].map(s =>
s
.replace(/[([^[]]*)]/g, '.$1.')
.split('.')
.filter(t => t !== '')
.reduce((prev, cur) => prev && prev[cur], from)
);
const obj = { selector: { to: { val: 'val to select' } }, target: [1, 2, { a: 'test' }] };
// Example
get(obj, 'selector.to.val', 'target[0]', 'target[2].a'); // ['val to select', 1, 'test']
14. Cách để gọi một hàm nào đó sau một khoảng thời gian (tính bằng mili giây)
const delay = (fn, wait, ...args) => setTimeout(fn, wait, ...args);
delay(
function(text) {
console.log(text);
},
1000,
'later'
);
// Logs 'later' after one second.
15. Cách để kích hoạt một sự kiện cụ thể trên một phần tử nhất định, tùy ý chuyển dữ liệu tùy chỉnh
const triggerEvent = (el, eventType, detail) =>
el.dispatchEvent(new CustomEvent(eventType, { detail }));
// Examples
triggerEvent(document.getElementById('myId'), 'click');
triggerEvent(document.getElementById('myId'), 'click', { username: 'bob' });
16. Cách để loại bỏ một even listener từ một phần tử
const off = (el, evt, fn, opts = false) => el.removeEventListener(evt, fn, opts);
const fn = () => console.log('!');
document.body.addEventListener('click', fn);
off(document.body, 'click', fn); // no longer logs '!' upon clicking on the page
17. Cách để có được định dạng có thể đọc được của số mili giây đã cho
const formatDuration = ms => {
if (ms < 0) ms = -ms;
const time = {
day: Math.floor(ms / 86400000),
hour: Math.floor(ms / 3600000) % 24,
minute: Math.floor(ms / 60000) % 60,
second: Math.floor(ms / 1000) % 60,
millisecond: Math.floor(ms) % 1000
};
return Object.entries(time)
.filter(val => val[1] !== 0)
.map(([key, val]) => `${val} ${key}${val !== 1 ? 's' : ''}`)
.join(', ');
};
// Examples
formatDuration(1001); // '1 second, 1 millisecond'
formatDuration(34325055574); // '397 days, 6 hours, 44 minutes, 15 seconds, 574 milliseconds'
18. Cách để tính số ngày giữa 2 ngày đã cho
const getDaysDiffBetweenDates = (dateInitial, dateFinal) =>
(dateFinal - dateInitial) / (1000 * 3600 * 24);
// Example
getDaysDiffBetweenDates(new Date('2021-06-17'), new Date('2021-06-20')); // 95
19. Cách để thực hiện một yêu cầu GET đến URL nào đó
const httpGet = (url, callback, err = console.error) => {
const request = new XMLHttpRequest();
request.open('GET', url, true);
request.onload = () => callback(request.responseText);
request.onerror = () => err(request);
request.send();
};
httpGet(
'https://jsonplaceholder.typicode.com/posts/1',
console.log
);
// Logs: {"userId": 1, "id": 1, "title": "sample title", "body": "my text"}
20. Cách để thực hiện một yêu cầu POST đến URL nào đó
const httpPost = (url, data, callback, err = console.error) => {
const request = new XMLHttpRequest();
request.open('POST', url, true);
request.setRequestHeader('Content-type', 'application/json; charset=utf-8');
request.onload = () => callback(request.responseText);
request.onerror = () => err(request);
request.send(data);
};
const newPost = {
userId: 1,
id: 1337,
title: 'Foo',
body: 'bar bar bar'
};
const data = JSON.stringify(newPost);
httpPost(
'https://jsonplaceholder.typicode.com/posts',
data,
console.log
);
// Logs: {"userId": 1, "id": 1337, "title": "Foo", "body": "bar bar bar"}
21. Cách để tạo một bộ đếm với phạm vi, bước đếm và thời lượng được chỉ định cho bộ chọn được chỉ định
const counter = (selector, start, end, step = 1, duration = 2000) => {
let current = start,
_step = (end - start) * step < 0 ? -step : step,
timer = setInterval(() => {
current += _step;
document.querySelector(selector).innerHTML = current;
if (current >= end) document.querySelector(selector).innerHTML = end;
if (current >= end) clearInterval(timer);
}, Math.abs(Math.floor(duration / (end - start))));
return timer;
};
// Ví dụ
counter('#my-id', 1, 1000, 5, 2000); // Tạo bộ đếm thời gian 2 giây cho phần tử id="my-id"
22. Cách để sao chép một chuỗi vào clipboard
const copyToClipboard = str => {
const el = document.createElement('textarea');
el.value = str;
el.setAttribute('readonly', '');
el.style.position = 'absolute';
el.style.left = '-9999px';
document.body.appendChild(el);
const selected =
document.getSelection().rangeCount > 0 ? document.getSelection().getRangeAt(0) : false;
el.select();
document.execCommand('copy');
document.body.removeChild(el);
if (selected) {
document.getSelection().removeAllRanges();
document.getSelection().addRange(selected);
}
};
// Ví dụ
copyToClipboard('Chuỗi ví dụ'); // 'Chuỗi ví dụ' đã được copy vào clipboard.
23. Cách để biết tab của trình duyệt của trang có đang được focus
const isBrowserTabFocused = () => !document.hidden;
// Ví dụ
isBrowserTabFocused(); // true
24. Cách để tạo một thư mục nếu nó không tồn tại
const fs = require('fs');
const createDirIfNotExists = dir => (!fs.existsSync(dir) ? fs.mkdirSync(dir) : undefined);
// Ví dụ
createDirIfNotExists('test'); // Tạo thư mục 'test', nếu nó không tồn tại
Tham khảo: https://dev.to/